Drawing Images Slowly
A Drawing of a Sunrise
Here is a program that draws some yellow lines on a blue background:
#include <allegro.h> int main() { if (allegro_init()!=0) return 1; set_color_depth(32); if (set_gfx_mode(GFX_AUTODETECT_WINDOWED, 800, 600, 0, 0)!=0) return 2; BITMAP* surface = create_bitmap(SCREEN_W, SCREEN_H); install_keyboard(); clear_to_color(surface, makecol(0,0,255)); for (int i=0;i<600;i+=5) line(surface,400,599,0,599-i,makecol(255,255,0)); for (int i=0;i<800;i+=5) line(surface,400,599,i,0,makecol(255,255,0)); for (int i=0;i<600;i+=5) line(surface,400,599,799,i,makecol(255,255,0)); blit(surface, screen, 0,0,0,0,SCREEN_W,SCREEN_H); while (!keypressed()) rest(20); return 0; } END_OF_MAIN()
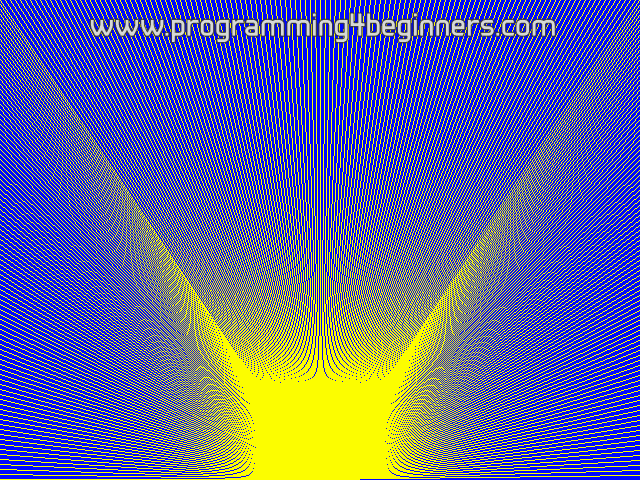
This program draws lines from the center of
the 'sun' towards the edges of the image. It contains three for
loops:
one for drawing lines towards the left edge, one towards the top edge, and one
for drawing lines towards the right edge.
We would now be interested in a program that does all this drawing more slowly so that we can observe how the image is created. To do that, the program should copy the drawing surface to the display surface after drawing each line, so that the newest change gets displayed. Then, we should make the program rest for a short time to slow it down. Here is the source code of the changed part:
clear_to_color(surface, makecol(0,0,255)); for (int i=0;i<600;i+=5) { line(surface,400,599,0,599-i,makecol(255,255,0)); blit(surface, screen, 0,0,0,0,SCREEN_W,SCREEN_H); rest(20); } for (int i=0;i<800;i+=5) { line(surface,400,599,i,0,makecol(255,255,0)); blit(surface, screen,0,0,0,0,SCREEN_W,SCREEN_H); rest(20); } for (int i=0;i<600;i+=5) { line(surface,400,599,799,i,makecol(255,255,0)); blit(surface, screen,0,0,0,0,SCREEN_W,SCREEN_H); rest(20); }
Make these changes, then run the program to observe the effect it produces.