6. Debugger
Step by Step
If you are using Code::Blocks IDE instead of Visual Studio, select Debug -> Step into (or press shift-F7) to start debugging. To advance the program to the next line, press the F7 key (instead of F10).
If a dialogue appears asking whether to build the program, choose Yes or hit the Enter key.
To start the debugger, press the F10 key or select Debug -> Step Over from the menu. The program will begin the execution, but then it will immediately be paused right at the brace (i.e. curly bracket) at the beginning of the function main
. A small yellow arrow pointing at the statement where the program has been paused will mark this line.
Every time you press the F10 key (do not do it yet), one line of the program will be executed. Press F10 key now. The yellow arrow will progress to the next line.

Should you press F10 key again, the statement currently being pointed at will get executed:
double sum=0;
Press the F10 key now. That will execute the statement pointed to and the yellow arrow will progress to the next line.
If you are using Code::Blocks IDE instead of Visual Studio, select Debug -> Debugging windows ->Watches to bring up the Watches window displaying local variables. You can then dock that window. To make Code::Blocks remember this arrangement, select View -> Perspectives -> save current.
During the process of debugging a program,
it is very useful to observe the values of particular variables. For example,
the value of the variable sum
can be observed in the Locals window in the lower left corner of your IDE.
In addition, it would be a good idea for the sake of this chapter to reduce the size of the IDE window so that the console window can be displayed alongside. This way you can keep track of the changes that will appear on standard output. You can arrange the two windows like this:
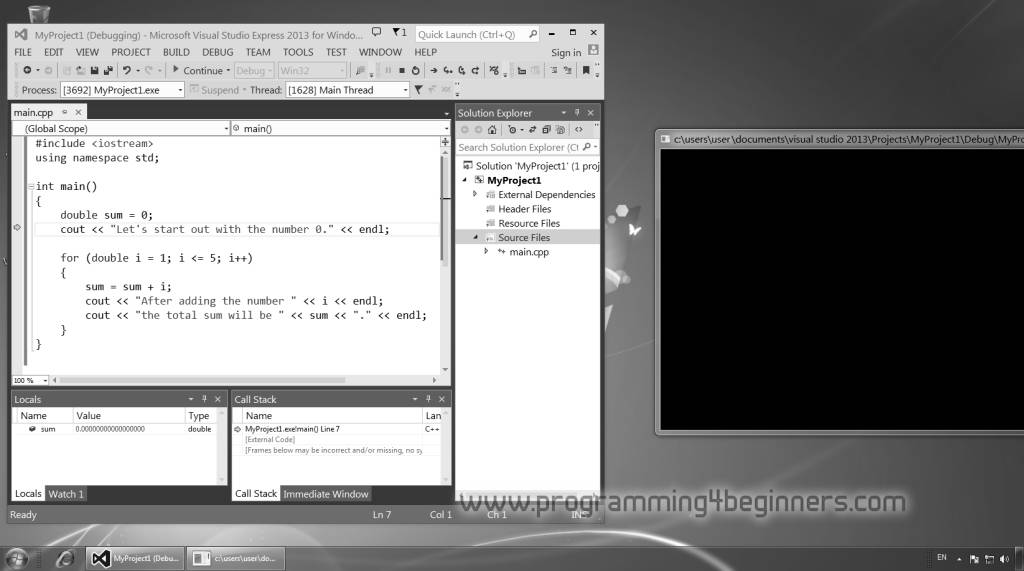
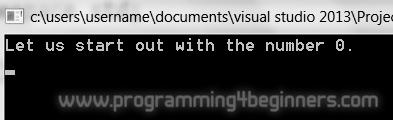
In the IDE, press F10 key again. As a result, another statement will get executed, and something will appear on the standard output.
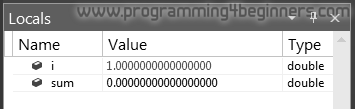
Press F10 key again. The Locals window will show that variable i
has assumed the value 1.
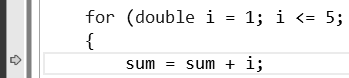
The yellow arrow should now point at the statement that will modify the variable sum
:
What will happen when you press F10 key again? First, have a look at the Locals window.
There you can see that the variable sum
equals
0, and that the variable i
equals 1. The statement that we are about to execute will add the
value of i
to the value of sum
and assign the result back to the variable sum
. Thus, the variable sum
should become equal to number 1 upon execution of this statement.
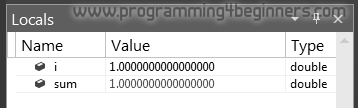
Indeed, after you press the F10 key again and look at the Locals window, the variable sum
will become equal to 1.
A red-colored text in the Locals window highlights the fact that the variable sum
has had its value modified.
You should now continue the execution of this program by repeatedly pressing the F10 key and keeping track of values of variables in the Locals window as well as the results of the program being printed out in the console window.
In Code::Blocks IDE, select Debug->Stop debugger or press the shift-F8 keys instead of F5 key).
Keep doing this until the yellow arrow points at the last closing brace at the end of the program. At this point, you should press F5 key to complete the execution of the program. Should you fail to do that, some strange files might open. Just press F5 key at that point, which will complete the execution.
Overall, by using a debugger we can examine the statement-wise execution of a program. You should have been able to notice the similarity of executing the program by hand and by a debugger.
Another useful scenario for deploying a debugger would be the case in which you are faced with a program whose behavior you do not fully understand - step by step execution often proves very helpful in clarifying the inner workings of a program.
Ultimately, the true purpose of a debugger is finding and removing errors in our programs, and those errors are colloquially called bugs by programmers; hence the name, debugger. We should point out that by bugs we do not refer to compile-time errors or syntactic errors as we have been calling them. That is because the compiler will be able to detect all such errors. We use the debugger for finding and exterminating a far more sinister type of errors that cannot be detected during the compilation process.