Animation and Keyboard
Time
There are several ways to keep track of
time in C++ programs. We could use the function time
from
the time.h
library. Unfortunately, it can only provide the time to the
precision of one second, which is not sufficient for displaying an animation.
This function is also rather old and might not function correctly after the year
2038, due to some issues.
The function clock
from
the same library can usually provide time intervals of higher accuracy. It is
sometimes quite simple and convenient to use this function. It would be our
second choice.
There are also functions from the C++ chrono library, which is rather new, but those are somewhat more complicated to use.
In our specific case, the simplest method to use is the Allegro's own mechanism for measuring time. It has the benefit of the time measurement pausing while the application is not in focus, which can be quite convenient.
In order to demonstrate how all that is supposed to work, here we present a program that displays a blue ball moving from left to right:
#include <allegro.h> int tickCount = 0; void MillisecondTick() { tickCount++; } int main() { if (allegro_init()!=0) return 1; set_color_depth(32); if (set_gfx_mode(GFX_AUTODETECT_WINDOWED, 800, 600, 0, 0)!=0) return 2; BITMAP* surface = create_bitmap(SCREEN_W, SCREEN_H); install_keyboard(); install_timer(); install_int(MillisecondTick, 1); while (!keypressed()) { int x = tickCount/10; clear_to_color(surface, makecol(255,255,255)); circlefill(surface,x,200,50,makecol(0,0,255)); blit(surface, screen, 0,0,0,0,SCREEN_W,SCREEN_H); rest(20); } return 0; } END_OF_MAIN()
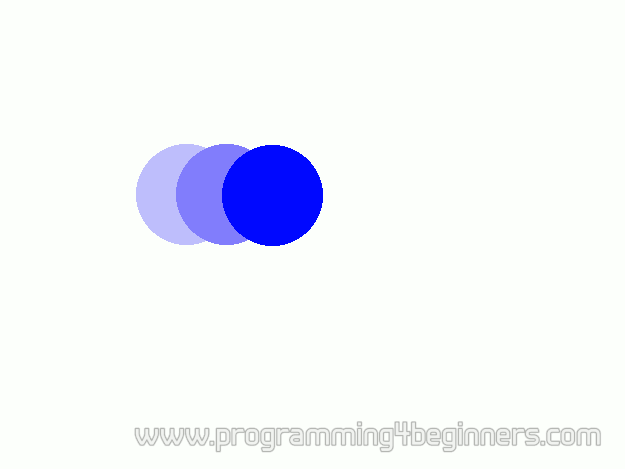
When you run this program, it will display a blue ball moving from left to right. If the focus is switched to another application (by pressing the keys Alt-Tab), the program will pause until it regains the focus.
The program can be ended at any
moment due to the condition checked at the beginning of the while loop. The
function keypressed
will yield truth when a key is pressed. The negation operator is
used to reverse that truth value, so that the while
loop executes while no key is
pressed.
The variable tickCount
has a value equal to the number of milliseconds elapsed since the start of the
program. A millisecond is a thousandth part of one second. Each millisecond the
function MillisecondTick
is called, causing the variable tickCount
to be increased by one. In
the while
loop, the position of the ball is stored in the variable named x
. The
value of this variable is set to tickCount/10
on every iteration of
the while loop. On every elapsed second, the tickCount
variable will be increased
by a thousand, so each second the variable x
will increase by 100, making the
speed of the ball equal to 100 pixels per second.
The ball is drawn and the whole image displayed on every iteration of the while loop, until the loop is aborted by pressing a key.
The statement:
install_timer();
tells the allegro library to enable the tracking of time. The following statement:
install_int(MillisecondTick, 1);
tells the Allegro library to call the MillisecondTick
function
each millisecond. But note that you should not use the install_int
function for other purposes except for incrementing the timer variable.